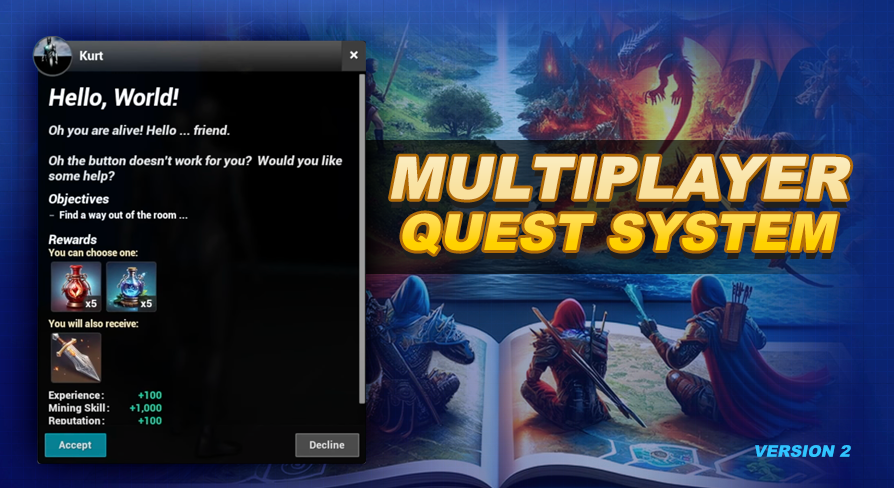
Quest Prerequisites
UE5 Quest System Version: 2.0What are Quest Prerequisites?
Quest Prerequisites are conditions that need to be fulfilled before a quest or its objectives become accessible to the player.
You can specify these prerequisites in the data table for each quest using the QuestPrerequisites map variable. In this map, each key represents a type of prerequisite, and the associated value provides the necessary details using our Prerequisite Syntax (see below).
Prerequisites are categorized into internal and external types. Internal prerequisites are checked within the quest system itself, verifying the state of other quests.
External prerequisites, on the other hand, use the Connected Systems BPI, enabling you to integrate conditions from other systems outside the quest system.
Prerequisite Syntax
Each prerequisite type can manage multiple conditions, which you delineate in the value field of the QuestPrerequisites map in the data table for your quest. For instance, if a prerequisite requires the completion of three specific quests, you'd choose Quest Complete as the key and set the value to quest1,quest2,quest3 using commas to separate each QuestRowName. For a single quest requirement, simply list that quest without any commas, like this: quest1
The prerequisite syntax also supports values, enabling you to define more detailed conditions. For example, if you're using an experience system and want a quest to require a minimum player level of 10, you'd choose the Player Level prerequisite with a value of: level:10
To set multiple conditions, like requiring a player level of 10 and a mining skill of 5, you'd format the value as: level:10,mining:5
The quest system will then parse this syntax and call your isQuestPrerequisiteComplete (part of the Connected Systems BPI) function separately for each condition:
- First call: PrerequisiteKey of level and a PrerequisiteValue of 10
- Second call: PrerequisiteKey of mining and a PrerequisiteValue of 5
Internal Prerequisites
Internal prerequisites rely on the state of other quests within the system and don't require extra setup. To define them, use the quest row name in the value syntax. If you need to specify multiple quests for a single prerequisite type, separate their names with a comma.
How External & Custom Prerequisites work ...
External & Custom prerequisite types are designed to work with the various Connected Systems in your game. To utilize custom prerequisites, you need to implement the isQuestPrerequisiteComplete function from the Connected Systems BPI in your player controller.
Your other systems also need to have the capability of exposing these values so you can evaluate them against the values the quest system sends to this function as inputs. For guidance on setting up and implementing this function, refer to the Connected Systems chapter in this documentation.
Once you've implemented the function, configure it to assess the inputs and return a boolean value indicating whether the custom prerequisite is met. The function receives three inputs: the Prerequisite Type (an enum), the Prerequisite Key, and the Prerequisite Value. The key and value are derived from your defined Syntax Value (see below).
The isQuestPrerequisiteComplete function is your one-stop method for evaluating all custom prerequisites. Use the Prerequisite Type enum to identify which specific prerequisite you are checking. These custom types are designed as generic placeholders to accommodate a range of common requirements in your game's quest system. To use them you will need to write the code to handle checking them against your other systems (we go over this in that Connected Systems chapter I keep mentioning).
In our demo world, we utilize custom prerequisites for both the quest and objective availability in the example with the light that turns on and off. This demonstrates how prerequisites can be effectively applied to individual objectives within a quest, adding depth and complexity to the gameplay experience.
In the background, this prerequisite utilizes the Custom type with a value of lightbulb. Within our handler (see demo world's player controller), we interpret this specific requirement to check the current state of our actor (in this case, a lightbulb) and if it's turned on, we return true; if it's off, we return false. The quest system takes care of the rest. Your role is simply to assess the condition based on the inputs provided and return a true or false value accordingly, informing the quest system whether the prerequisite is met.
List of External Prerequisite Types
If you would like examples of most of these functions in action have a look at the Connected Systems page for a run through using my Inventory and Experience Systems.
Objective Prerequisites
Just as with quests, Quest Objectives can also be set with prerequisites, allowing you to control when an objective can be completed. This feature enables you to create a more dynamic and interdependent quest structure where the completion of certain objectives might depend on various conditions being met.
Reevaluating Availability
One of the Quest Options is Reevaluate Availability?. When this is set to true, the system will periodically reassess the prerequisites for a quest that is currently marked as available.
If, upon reevaluation, the prerequisites are not met, this option will cause the Quest State to switch back to 'Not Available.' This ensures that the quest's availability accurately reflects the current state of its prerequisites at all times.